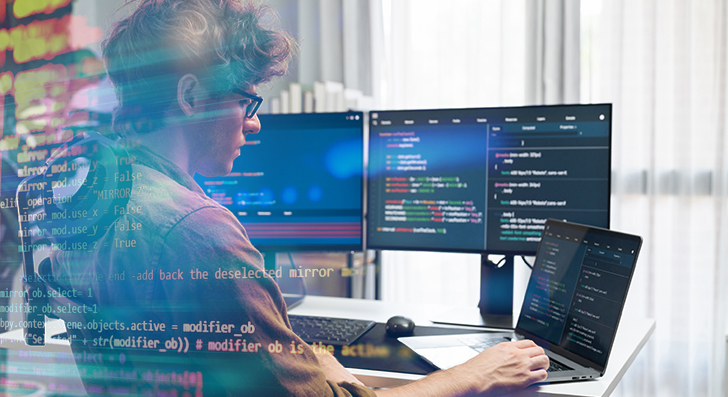
Scalability implies your application can tackle advancement—far more consumers, more details, plus more targeted visitors—with no breaking. Like a developer, constructing with scalability in mind will save time and tension afterwards. Listed here’s a transparent and functional manual to help you start by Gustavo Woltmann.
Style and design for Scalability from the Start
Scalability just isn't some thing you bolt on afterwards—it should be aspect of one's system from the start. Many apps fail whenever they grow rapidly because the initial design can’t take care of the extra load. Being a developer, you need to Consider early regarding how your program will behave stressed.
Start by planning your architecture to generally be flexible. Avoid monolithic codebases in which all the things is tightly connected. As an alternative, use modular structure or microservices. These patterns split your application into lesser, impartial pieces. Every module or provider can scale By itself without the need of affecting The entire technique.
Also, give thought to your databases from day just one. Will it have to have to handle a million consumers or maybe 100? Choose the ideal kind—relational or NoSQL—dependant on how your data will develop. Program for sharding, indexing, and backups early, Even though you don’t need to have them still.
A further important point is to prevent hardcoding assumptions. Don’t produce code that only is effective under current circumstances. Take into consideration what would take place Should your consumer base doubled tomorrow. Would your app crash? Would the database slow down?
Use style patterns that assistance scaling, like message queues or event-pushed units. These assistance your application handle more requests without getting overloaded.
Whenever you build with scalability in your mind, you are not just planning for achievement—you're reducing potential headaches. A well-prepared technique is simpler to maintain, adapt, and mature. It’s superior to get ready early than to rebuild later.
Use the best Database
Deciding on the suitable database is a vital Section of creating scalable applications. Not all databases are crafted precisely the same, and utilizing the Incorrect you can sluggish you down or even induce failures as your app grows.
Start by knowledge your info. Can it be hugely structured, like rows in the table? If Of course, a relational database like PostgreSQL or MySQL is a great suit. These are solid with relationships, transactions, and regularity. They also guidance scaling tactics like study replicas, indexing, and partitioning to manage much more website traffic and information.
If the information is a lot more flexible—like consumer exercise logs, merchandise catalogs, or documents—take into account a NoSQL possibility like MongoDB, Cassandra, or DynamoDB. NoSQL databases are far better at managing big volumes of unstructured or semi-structured knowledge and will scale horizontally a lot more quickly.
Also, think about your browse and create designs. Are you presently carrying out numerous reads with much less writes? Use caching and read replicas. Have you been managing a major create load? Take a look at databases that may take care of significant write throughput, or perhaps function-dependent details storage systems like Apache Kafka (for short-term knowledge streams).
It’s also clever to Believe forward. You may not need to have State-of-the-art scaling options now, but choosing a database that supports them indicates you won’t want to change later on.
Use indexing to hurry up queries. Stay clear of unnecessary joins. Normalize or denormalize your data based on your access patterns. And usually keep track of database overall performance as you develop.
Briefly, the appropriate databases is dependent upon your app’s construction, speed requirements, and how you expect it to grow. Choose time to select wisely—it’ll help you save loads of hassle later on.
Enhance Code and Queries
Quick code is key to scalability. As your application grows, just about every modest delay adds up. Improperly written code or unoptimized queries can decelerate effectiveness and overload your technique. That’s why it’s crucial that you Develop efficient logic from the beginning.
Start off by creating clean, very simple code. Prevent repeating logic and take away anything at all pointless. Don’t pick the most intricate Remedy if a straightforward just one operates. Keep your features brief, concentrated, and simple to test. Use profiling applications to seek out bottlenecks—locations where by your code normally takes as well extensive to run or takes advantage of excessive memory.
Subsequent, check out your database queries. These often sluggish things down in excess of the code itself. Ensure that Every question only asks for the data you really have to have. Keep away from SELECT *, which fetches almost everything, and as an alternative find certain fields. Use indexes to hurry up lookups. And avoid undertaking a lot of joins, Particularly throughout significant tables.
Should you detect exactly the same knowledge remaining asked for many times, use caching. Shop the outcome quickly using resources like Redis or Memcached therefore you don’t have to repeat pricey operations.
Also, batch your databases functions after you can. Rather than updating a row one after the other, update them in teams. This cuts down on overhead and makes your app a lot more productive.
Make sure to exam with large datasets. Code and queries that function fantastic with one hundred data could crash every time they have to deal with 1 million.
In a nutshell, scalable apps are rapidly applications. Maintain your code restricted, your queries lean, and use caching when wanted. These techniques assistance your software continue to be sleek and responsive, at the same time as the load raises.
Leverage Load Balancing and Caching
As your app grows, it has to handle much more customers and much more site visitors. If every little thing goes by means of a single server, it's going to swiftly become a bottleneck. That’s exactly where load balancing and caching come in. These two resources assist keep your application rapid, steady, and scalable.
Load balancing spreads incoming targeted traffic across numerous servers. Instead of one server accomplishing the many get the job done, the load balancer routes end users to unique servers determined by availability. This implies no single server receives overloaded. If one particular server goes down, the load balancer can deliver traffic to the Some others. Equipment like Nginx, HAProxy, or cloud-primarily based solutions from AWS and Google Cloud make this straightforward to put in place.
Caching is about storing information quickly so it could be reused speedily. When consumers ask for the exact same details again—like an item web page or simply a read more profile—you don’t should fetch it from your databases whenever. You are able to provide it in the cache.
There's two prevalent varieties of caching:
one. Server-aspect caching (like Redis or Memcached) suppliers knowledge in memory for fast entry.
2. Consumer-facet caching (like browser caching or CDN caching) retailers static data files near to the person.
Caching minimizes databases load, enhances velocity, and helps make your application much more successful.
Use caching for things that don’t adjust often. And constantly make sure your cache is up to date when details does alter.
Briefly, load balancing and caching are easy but strong applications. Alongside one another, they help your app cope with more consumers, continue to be fast, and Recuperate from challenges. If you propose to develop, you may need both.
Use Cloud and Container Resources
To develop scalable purposes, you need instruments that permit your application develop very easily. That’s wherever cloud platforms and containers are available. They offer you flexibility, minimize setup time, and make scaling Considerably smoother.
Cloud platforms like Amazon Website Services (AWS), Google Cloud Platform (GCP), and Microsoft Azure let you rent servers and providers as you may need them. You don’t have to purchase hardware or guess long term capability. When site visitors will increase, it is possible to insert additional methods with just a couple clicks or mechanically applying vehicle-scaling. When targeted visitors drops, you could scale down to economize.
These platforms also give solutions like managed databases, storage, load balancing, and security tools. You can focus on building your application in place of taking care of infrastructure.
Containers are One more crucial Instrument. A container packages your application and anything it should run—code, libraries, settings—into a person device. This can make it effortless to move your application involving environments, from the laptop to the cloud, devoid of surprises. Docker is the most well-liked Instrument for this.
Once your application makes use of multiple containers, applications like Kubernetes allow you to control them. Kubernetes handles deployment, scaling, and Restoration. If just one element of the application crashes, it restarts it mechanically.
Containers also allow it to be straightforward to individual elements of your application into providers. You can update or scale sections independently, which can be perfect for efficiency and trustworthiness.
In a nutshell, using cloud and container instruments indicates you could scale quickly, deploy conveniently, and Get better swiftly when complications take place. If you prefer your app to improve with out boundaries, begin employing these tools early. They preserve time, cut down threat, and make it easier to stay focused on making, not fixing.
Check Anything
In the event you don’t observe your application, you gained’t know when factors go wrong. Monitoring aids the thing is how your application is performing, spot troubles early, and make improved decisions as your app grows. It’s a crucial Component of building scalable methods.
Commence by monitoring primary metrics like CPU use, memory, disk space, and response time. These inform you how your servers and expert services are doing. Tools like Prometheus, Grafana, Datadog, or New Relic will help you acquire and visualize this knowledge.
Don’t just watch your servers—observe your application much too. Keep an eye on how long it takes for customers to load webpages, how often mistakes occur, and in which they take place. Logging equipment like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly can assist you see what’s taking place inside your code.
Put in place alerts for significant challenges. One example is, If the reaction time goes previously mentioned a limit or perhaps a services goes down, you need to get notified instantly. This helps you take care of challenges rapid, generally ahead of consumers even recognize.
Monitoring is usually handy if you make adjustments. In the event you deploy a new function and find out a spike in glitches or slowdowns, you'll be able to roll it back right before it will cause actual harm.
As your application grows, targeted traffic and information maximize. Devoid of monitoring, you’ll pass up signs of trouble right until it’s way too late. But with the proper applications in place, you continue to be in control.
In short, monitoring helps you maintain your app reliable and scalable. It’s not almost spotting failures—it’s about knowledge your method and ensuring that it works very well, even under pressure.
Closing Thoughts
Scalability isn’t just for significant firms. Even small apps have to have a powerful Basis. By designing thoroughly, optimizing wisely, and using the ideal resources, you may Develop applications that mature easily devoid of breaking under pressure. Start out small, Feel significant, and Develop sensible.